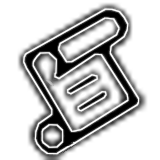
Write your code through Instantiable Constructors
8388 words, 31 min read
Donate to authorWhen attempting to write a game outside of a game engine, one would start with an empty text file to create an overarching application loop within their programming language of choice — that is, how the application keeps on repeating to not finish its execution after a single iteration and how and when events of each object and concept within are allowed to be executed. Game engines tend to achieve this through their own event systems, which is represented in GameMaker through Object Events. What they really are is code executing another code at specific timing. However, GameMaker does not offer precise control over this timing and it can depend on unpredictable things, such as the alphabetical order of Object Asset names.
This guide is about writing a custom framework using GameMaker Language for executing object code to gain full control of the process. Doing so benefits all kinds of GameMaker projects, both simple and complex, with little actual drawbacks. Effects range from enhancing the programming experience by working with a transparent and understandable code, to extending control over timing of execution and display of elements within the application, enabling several state of the art graphical and networking paradigms.
This guide will go through the setup of such approach, its example benefits, as well as ideas for advanced use of presented concepts. The setup is kept simple, as not many assets are involved in primary functionality.
Techniques presented below are dependent on following GameMaker systems:
- Objects, Instances, Object Events, Event Order, Variables and Variable Scope, Built-In Instance Variables, Instance Keywords and Parent Objects.
- Functions and Method Variables.
- Structs and Constructors, Static Variables and Garbage Collection.
- Data Types, Arrays and Priority Queues.
- Rooms, Room Order, Room Creation Code, Layers and Sprites.
- Asset Editors and Code Regions.
These concepts are supported in runtimes after the 2.3 version of GameMaker Studio 2, released in August 2020.
The Concept
GameMaker only supports performing the application loop using Objects, but this can be reduced to just one object acting as the Event Queue and a handful of supporting Blueprint Objects for use with features exclusive only to them. Everything else can be then operated through Instantiable Constructors and constructors inheriting Instantiable Constructors. This hierarchy works as follows:
- Event Queue: Only one is used. Manages the application loop to execute repeated Events submitted by all instanced constructors into Priority Queues, in order according to priority they were assigned.
- Blueprint Objects: Several are used, their amount depends on the needs of the project. Each has a different set of Events of two types: Repeated (
Step
,Draw
,Draw GUI
Events) and instant (every other Event). Their Instances execute repeated Events to submit Event Methods of constructors that instantiated them to the Event Queue, for it to execute them at appropriate time. Instant Events are executed by Instances at the moment they would normally happen. - Instantiable Constructors: Exactly as many as the number of Blueprint Objects used. They create and operate Blueprint Objects from the level of a constructor that inherits them, supplying them with methods used to operate them, such as actually creating and destroying their instances.
- Constructors inheriting Instantiable Constructors: As many as the project requires are used. This is what the code of the actual project will be contained in after this framework is set up. Each of these constructor inherits an Instantiable Constructor of appropriate type to inherit methods executed during specific Object Events as necessary. Their methods with names set up in an Instantiable Constructor they inherit are the Event Methods partaking in this system.
Benefits
- Increased similarity to programming without game engine tools, making use of ubiquitous knowledge that is easier to transfer between programming environments:
- Direct control over order and timing of execution and rendering:
- Simplifies executing Events outside of their usual order when necessary, using features exclusive to methods.
- Enables the possibility of supporting high screen refresh rates, provided the code of object logic is properly separated from rendering code.
- Can be used in networking to control Event execution in respect to connection delay.
- Allows manipulating input through variables without causing additional input delay.
- Reduces reliance on systems built into GameMaker, offering a choice between actively using them or keeping their usage to minimum:
- Enables the possibility of creating the project using just one Layer, as render ordering is handled by order of Event execution, and using just one Room, as Instance management is handled with code.
- Works on Object code in a namespace without Built-In Instance Variables, as constructors do not use them. This prevents accidental usage of systems based on these variables, as Instance reference has to be explicitly used in constructor code.
- Encourages lesser need of individual Objects for features, which can be operated by manager constructors. Constructors are memory-efficient, as their content is entirely customizable.
- Introduces additional convivence to the process of programming:
Implementation
Event Queue
The first concept to introduce is the Event Queue, through which repeated Events will be executed:
- Create a new Object Asset named
EventQueue
and enable its properties:Visible
andPersistent
. Then, add following Events to it:Create
,Clean Up
,Step
,Draw
andDraw GUI
. - Ensure a single instance of
EventQueue
is created when the application is launched. This can be done in several different ways using a Room Asset that is the first in the Room Order:- Edit Room Creation Code to create a Layer, on which an instance of
EventQueue
will be created by callinginstance_create_layer()
. - Edit Room Creation Code to create an individual Layer, on which an instance of
EventQueue
will be created at specified depth by callinginstance_create_depth()
. - Use the the Room Editor to create or select an existing Instance Layer and drag the
EventQueue
asset into it using the cursor.
- Edit Room Creation Code to create a Layer, on which an instance of
- In
Create
Event ofEventQueue
, add code declaring a variable with a Priority Queue for each type of a repeated Event:
step = ds_priority_create(); draw = ds_priority_create(); drawGUI = ds_priority_create();
- In
Step
,Draw
andDraw GUI
Events, add code iterating through above Priority Queues, using the following pattern. In each Event, change the name of thequeue
variable to match an appropriate Priority Queue for the current Event:
repeat (ds_priority_size(queue)) { var _event = ds_priority_delete_min(queue); with (_event[0]) { with (_event[0]) { _event[1](); } } }
Explanation:
- When instances of Blueprint Objects execute their repeated Event, they add a value to appropriate Priority Queue of
EventQueue
. That value is added at the priority assigned to Instantiable Constructor, which created that instance. It decides the order of execution. The value is an array containing two entries: the reference to that constructor and its Event to execute. - The
with
statement is used twice to ensure proper scope of execution from within the Event Method code, both changing the scope to the constructor. The first use of this statement sets theother
scope, so it does not refer toEventQueue
and second sets theself
scope. - Once scopes are set, the Event is executed, then it is removed from the Priority Queue and iteration repeats until it is empty.
- In
Clean Up
Event, add code freeing up memory used byEventQueue
in case it is destroyed, to prevent memory leaks from occurring:
ds_priority_destroy(step); ds_priority_destroy(draw); ds_priority_destroy(drawGUI);
Instantiable Constructor Parent
Next is the setup of primary constructor, from which all Instantiable Constructors will inherit. It contains the framework for Event Methods, execution priorities and methods used to manage Instances .
- Create a new Script Asset named
Instance
, then edit it to addconstructor
after its name. - In the code of this newly made constructor, add following static properties:
static object = Object; static createEvent = function() {} static stepEvent = function() {} static drawEvent = function() {} static cleanUpEvent = function() {}
Explanation:
- The property
object
is the Blueprint Object that will be used for this Instantiable Constructor. The value of this property will be replaced in Instantiable Constructors inheriting this constructor. - The Event Methods reference empty methods, which will become the default content of each Event. Each constructor inheriting an Instantiable Constructor can have static properties with these names to declare content of their own Events.
- Add a method that will return a struct with variables added to instance of the
object
of this constructor. At minimum, it needs to contain a reference to the constructor:
static getBaseInstanceData = function() { var _base = self; return { base: _base }; }
- Declare a
priority
property and assign any integer number to it. That will become the default priority for any constructor inheriting this one, which then can be overridden in their own constructor declarations. This number decides which Instance executes its repeated Events first, in cases where that order matters. With howEventQueue
was set up in previous steps, the lower this number is, the earlier its Event executes, suggesting that0
can be considered the lowest number used for priorities. - Add a
createInstance()
method that will instantiate theobject
of this constructor and add variables to it, as returned bygetBaseInstanceData()
. Exact contents of this method are flexible, depending on the rest of the project, as it can be used to expand the Instance with additional features or configure its Built-In Variables by adding them upon creation. A Layer needs to be exist in the application at this point to contain the Instance. Following example code assumes one exists as ainstanceLayer
variable ofEventQueue
:
static createInstance = function() { instance_create_layer(0, 0, EventQueue.instanceLayer, object, self.getBaseInstanceData()); }
- Add
destroyInstance()
method that will destroy the instance of this constructor, set its reference toundefined
and returnundefined
, so the call of this method can be assigned to variable referencing this constructor, removing reference to it. Removing all references to this constructor will allow the Garbage Collector to free memory it occupied after it can be no longer used:
static destroyInstance = function() { instance_destroy(instance); instance = undefined; return undefined; }
Blueprint Object
As the above Instantiable Constructor relies on an object
property to function, it must be created with the same asset name it references to.
- Create a new Object Asset named
Object
and enable itsVisible
property. Then, add following Events to it:Create
,Clean Up
,Step
andDraw
. - In
Create
Event, add code declaringbase.instance = id;
. Thebase
variable is a reference to the constructor that created this Instance. This is the earliest timing at which the Instance Reference can be assigned in it, making it possible to access it from theCreate
Event Method in the Instantiable Constructor code that is about to be executed. - Below above code, add the following code executing the
Create
Event Method in the scope of the Instantiable Constructor. As it is an instant Event, it needs to be executed immediately:
with (base) { createEvent(); }
- Add Event Method execution with the above code pattern to the
Clean Up
Event by replacingcreateEvent()
withcleanUpEvent()
. - In
Step
andDraw
Events, refer toEventQueue
and add an array withbase
reference and an appropriate Event Method, according to following code pattern, wherequeue
needs to be replaced with the appropriate variable referencing its Priority Queue for the current Event:
ds_priority_add(EventQueue.queue, [base, stepEvent], base.priority);
Examples
Time tracker
This example illustrates a single-purpose constructor inheriting an Instantiable Constructor that needs to execute each Step
Event to operate. Normally, it would need to be fit into code of another Object to be continuously executed, but for an Instantiable Constructor it is possible to operate on its own after simply being constructed anywhere in the project after EventQueue
became operational.
function Time() : Instance() constructor { priority = 1; #region [Events] static createEvent = function() { stepCount = 0; } static stepEvent = function() { ++stepCount; } #endregion createInstance(); }
An Instantiable Constructor like this should be declared with a low priority, meaning it will be one of the earliest to execute, so Instantiable Constructors executing later can access its updated information.
A feature Object Assets have is that they can be referred to directly, which is intended for use with Singleton Objects, where only one Instance is created. That makes it possible to refer ObjectName.variableName
from code as long as it its Instance exists. This behavior can be reproduced for any kind of variable by using globalvar
. This way, any keyword that is not already in use can be registered as a globally accessible variable, without the need of the global.
prefix. Instead, its name is written right after the globalvar
keyword exactly once in the code base. Then, that registered variable can have values set to and read from it, in separate calls from its initial declaration.
Moving and colliding instance
Following constructor operates simple code responsible for rendering, movement and collision. To execute it, it can be constructed twice anywhere in the project after EventQueue
became operational.
function ExampleMovingInstance() : Instance() constructor { priority = 15; #region [Events] static createEvent = function() { static identifier = 0; ++identifier; movementSpeed = ((identifier == 1) ? 5 : 0); instance.x = round(window_get_width() * (0.325 * identifier)); instance.y = round(window_get_height() * 0.5); instance.sprite_index = self.createSprite(50); } static stepEvent = function() { var _speed_x = ((keyboard_check(ord("D")) - (keyboard_check(ord("A")))) * movementSpeed); var _speed_y = ((keyboard_check(ord("S")) - (keyboard_check(ord("W")))) * movementSpeed); var _collision_x = sign(_speed_x); var _collision_y = sign(_speed_y); repeat (abs(_speed_x)) { if (!self.isColliding(_collision_x)) { instance.x += _collision_x; } else { break; } } repeat (abs(_speed_y)) { if (!self.isColliding(undefined, _collision_y)) { instance.y += _collision_y; } else { break; } } } static drawEvent = function() { with (instance) { draw_self(); } } #endregion #region [Methods] static isColliding = function(_distance_x = 0, _distance_y = 0) { with (instance) { return (collision_rectangle((bbox_left + _distance_x), (bbox_top + _distance_y), (bbox_right + _distance_x), (bbox_bottom + _distance_y), base.object, false, true) != noone); } } static createSprite = function(_size) { var _surface = surface_create(_size, _size); surface_set_target(_surface); draw_clear(c_white); surface_reset_target(); var _sprite = sprite_create_from_surface(_surface, 0, 0, _size, _size, false, false, (_size * 0.5), (_size * 0.5)); surface_free(_surface); return _sprite; } #endregion createInstance(); }
Explanation:
- The priority of the instance is declared. This will cause the repeated Events of this constructor to execute after ones using the default priority value. This step is optional.
- A
createEvent()
method is declared. Just having this method assigned to a property of this particular name will cause it to be executed whencreateInstance()
is called. The code of the method sets property of a constructor in accordance to a declared static property, which is a feature not available to Object code normally. After that, an instance is referred to configure its Built-In Instance Variables. - A
stepEvent()
method is declared, which will be added intoEventQueue
eachStep
Event. Its code depends on method of the constructor declared below it to operate collision in a loop as a part of its movement code. - A
drawEvent()
method is declared, which will be added intoEventQueue
eachDraw
Event, as long as this Instance has itsvisible
Built-In Variable set totrue
. The scope must be changed to executedraw_self()
, as it is an object-specific function. It can be replaced withdraw_sprite()
for use without changing scope. - Two constructor methods are declared. One to be used in the
Step
Event in operating collisions through theinstance
scope and another to generate a Sprite assigned during creation. - The
createInstance()
method is called. This will immediately create the Instance. Calling this method can be omitted if a constructor is meant to be only constructed, but instantiated later.
According to the implementation of EventQueue
, the instance
property is referred to and set as scope multiple times in above example. Hence, this programming paradigm is most effective when designed with manager constructors, which would use non-instance constructors to operate logic of each concept and render all results in one go. However, if it is a necessity for the project, the EventQueue
can be also set up to instead execute in instance
scope, in which case its base
Instantiable Constructor will become what will need to be specifically referenced.
Expanding Implementation
After the initial setup, different kinds of Blueprint Objects can be added into the project as they become necessary. Object Assets must have their Events added manually, for their use to be possible with Instantiable Constructors. While it could be handled by just creating one Object Assets with code for every kind of an Event supported by GameMaker, it usually is not necessary. This is also the case for the Begin
and End
variants of repeated Events, since the priority system acts as a replacement in cases where such distinction is not required to use functionality relying on it. Creating specific Blueprint Objects communicates the intent of each constructor inheriting an Instantiable Constructor. For example, stating that a constructor inherits InterfaceInstance
constructor makes it immediately clear it was made to access the Draw GUI
Event, which the Instance
constructor does not operate.
Instructions below exemplify an implementation of said Blueprint Object and its respective Instantiable Constructor by inheriting from Instance
constructor, so it can be inherited further by constructors specific to each project:
- Create a new Object Asset named
InterfaceObject
, enable itsVisible
property and setObject
as its Parent Object. Then, addDraw GUI
Event to it. - Copy the code of
Draw
Event fromObject
and paste it into the newly createdDraw GUI
Event, then change the uses of thedraw
Priority Queue todrawGUI
. - Create a new Script Asset named
InterfaceInstance
, then edit it to make it contain the following code:
function InterfaceInstance() : Instance() constructor { static object = InterfaceObject; static drawGUIEvent = function() {} }
Explanation: 4. This constructor is made an Instantiable Constructor by inheriting the Instance
constructor, which causes it to also contain setup necessary for it to function that way. 5. The object
property has to be assigned to the new Blueprint Object, which in this case is the InstanceObject
. 6. The Instance
constructor does not contain drawGUIEvent
, so it has to be added as a static property referencing an empty method.
Implementing Advanced Features
With the use of Event Methods, it becomes possible to support advanced techniques that require full control of the execution timing. This section presents an implementation of high refresh rate support. While it is a complicated topic in general, it will serve as a simplified example of what can be achieved by manipulating execution loop of the application.
The default configuration of a newly created GameMaker project sets it to execute at 60 frames per second, which is expressed in intent of executing repeated Events at most of 60 times during that second. This value ensures every export target is able to universally display the graphics of the same application. However, it does not make full use of devices that support refresh rates higher than 60, which would possibly allow them to achieve smoother visual motion and more responsive input.
Note that to see the intended result of this section, it must be tested on a display with a refresh rate of more than 60. The operating system also needs to be configured to use the exact refresh rate that is being tested — setting lower frame per second value than the current refresh rate will introduce graphical tearing and higher frame rates will become unusable, as GameMaker runtime will not execute its application loop in a higher frame-per-second rate than the refresh rate of the screen.
Following implementation assumes the intent of executing the Step
Event code exactly the same for every kind of a device, but executing the Draw
and Draw GUI
Events at the highest refresh rate available. If the refresh rate is higher than 60, an appropriate number of Step
Events will be skipped, while all Draw
and Draw GUI
Events will execute with speed of the motion of graphics divided by the ratio of skipped Step
Events. This will allow the screen to display more frames of motion in the same amount of real time. For this to work properly, the architecture of the project must keep a clear separation between logic of each Instance in Step
Events and their displayed result in Draw
and Draw GUI
Events.
In code from implementation above, perform the following:
- Edit the code of
EventQueue
. In theCreate
Event, declarecycle = 0;
and replace the code of itsStep
Event with the following code:
cycle += (1 / (max(60, game_get_speed(gamespeed_fps)) div 60)); if (cycle >= 1) { repeat (ds_priority_size(step)) { var _event = ds_priority_delete_min(step); with (_event[0]) { with (_event[0]) { _event[1](); } } } cycle = 0; } else { ds_priority_clear(step); }
Explanation:
- This code divides the execution of
Step
Event Methods into cycles. TheStep
Event Methods will be executed only during a completion of a cycle. Otherwise, their requests will be discarded by clearing thestep
Priority Queue. - If the target number of frames per second is
60
or less, theStep
Event Methods will always be executed. Otherwise, thatStep
Event will be only a part of the cycle. The more time the target number of frames per second can be divided by60
, the lesser part of a single cycle it makes.
- Add
game_set_speed(display_get_frequency(), gamespeed_fps);
to anyStep
Event in the project, such as the at the beginning of theStep
Event ofEventQueue
. This will update the target frame per second number to the highest value handled by the operating system. - Edit the
ExampleMovingInstance
constructor to declarepreviousLocation = [instance.x, instance.y];
at the end of itscreateEvent
and at the start of itsstepEvent
. Then, replace itsdrawEvent
code with the following:
draw_sprite(instance.sprite_index, instance.image_index, lerp(previousLocation[0], instance.x, EventQueue.cycle), lerp(previousLocation[1], instance.y, EventQueue.cycle));
After launching the project, the movement of the example moving instance should appear smoother on displays with refresh rate of 120 and higher, as its movement is now interpolated during additional Draw
Events to target location set during each Step
Event executing at the end of the cycle. This is the simplest example of this concept.
Afterword
This concludes the introduction to concepts of GML Instantiable Constructors.
An importable project file is available. It contains minimal setup, examples and does not rely on dependencies.
A repository with an extended version of this framework is available, with its up to date documentation. It will be updated periodically with new features, corrections and further Blueprint Objects and their Instantiable Constructors.